This sample source code below shows how to implement Gaussian blur filter in OpenGL (WebGL) shading language. This is a popular algorithm in computer graphics that results in a blurry image.
Interactive Preview: Online Image Blur
Source Code
The GL shader code below implements a separable convolution that we can use for a Gaussian filter image blur effect. The GSLS code convolve a one-dimensional kernel with coefficients once row-wise and once column-wise.
precision mediump float;
// our texture
uniform sampler2D u_image;
#define KERNEL_SIZE 15
uniform vec2 u_textureSize;
uniform int u_direction;
uniform float u_kernel[KERNEL_SIZE];
void main() {
vec2 textCoord = gl_FragCoord.xy / u_textureSize;
vec2 onePixel = ((u_direction == 0) ? vec2(1.0, 0.0) : vec2(0.0, 1.0)) / u_textureSize;
vec4 meanColor = vec4(0);
int ms = KERNEL_SIZE / 2;
for (int i = 0; i < KERNEL_SIZE; i++)
{
meanColor += texture2D(u_image, textCoord + onePixel*vec2(i - ms))*u_kernel[i];
}
gl_FragColor = meanColor;
}
The code accepts the following shader variables:
- u_image – this is our source image
- u_kernel – the filtering Gaussian kernel as one-dimension floating point array
- u_direction – the filter direction – horizontal or vertical
How it works?
The algorithm applies a smoothing effect to images using a Gaussian filter.
Let’s say we have an image source ready as an OpenGL/WebGL texture. The following steps then briefly describe how the algorithm works:
- Initially we must calculate a Gaussian kernel
- We then assign it to the uniform variable u_kernel
- Set the uniform parameter u_direction – rows or columns
- Execute the shader program for both directions
Example Results
The following picture depicts an example output from the source code:
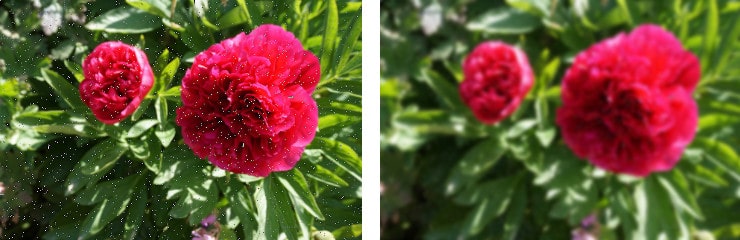
Bonus Tip
In case you are looking for a pure Java Script solution then you may take a look at the Gaussian blur in plain JS. You can also take a look at how to apply various filters via SVG.
Try it Online
You can explore the above algorithm in our free online image processing app.